Over the past few weeks I haven’t worked on Speakers Corner so much, but recently I’ve followed a lynda tutorial and have successfully created the functionality for adding and removing data to firebase through the site. At the moment it adds and removes meeting names and displays them on the site but I’ll post the code to show how I’ll be changing this to fit my site.
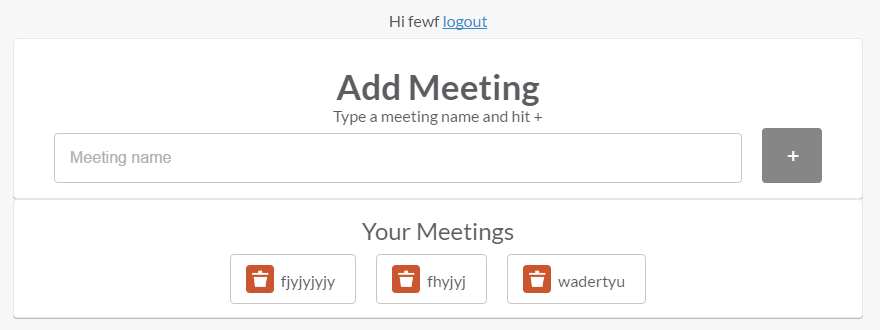
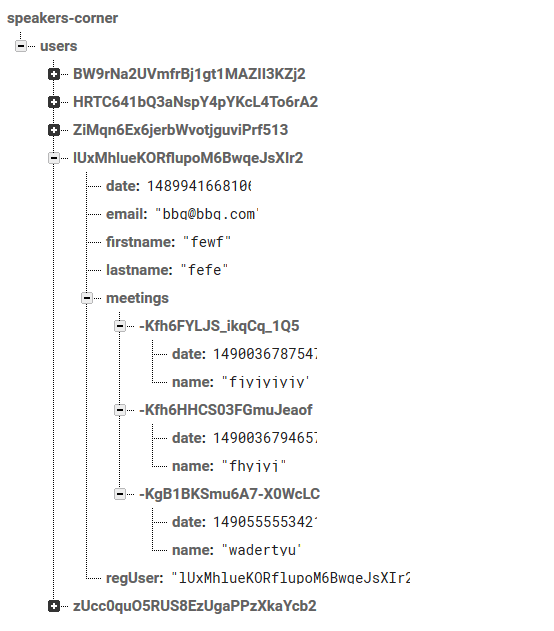
meetings.js
myApp.controller(‘MeetingsController’ ,
[‘$scope’, ‘$firebaseAuth’, ‘$firebaseArray’,
function($scope, $firebaseAuth, $firebaseArray) {
var ref = firebase.database().ref();//reference to the firebase database
var auth = $firebaseAuth(); //uses the authentication service and places it in the auth variable
auth.$onAuthStateChanged(function(authUser) {//uses a method to run a function when a user logs in, which returns true and is stored in the authUser variable
if(authUser) { //if authUser exists run the following:
var meetingsRef = ref.child(‘users’).child(authUser.uid).child(‘meetings’);//goes to users/(userid)/meetings in firebase
var meetingsInfo = $firebaseArray(meetingsRef);//pulls an array of info from the variable ‘meetingsRef’
$scope.meetings = meetingsInfo; //puts meetingsInfo into meetings
$scope.addMeeting = function() {
meetingsInfo.$add({ //$add is how to push data to the database
name: $scope.meetingname, //pushes the input field meetingname from meeting.html
date: firebase.database.ServerValue.TIMESTAMP //pushes the server time
}).then(function() {
$scope.meetingname=”;//clears the input from the meetingname feild
});//promise
}//addmeeting
$scope.deleteMeeting = function(key) {
meetingsInfo.$remove(key); //$remove is how to delete things from the database
}
}//Authuser
});//onAuthStateChanged
}]); //myApp.controller |
Meetings.html
<div class=”card meetings cf”>
<div class=”textintro”>
<h1>Add Meeting</h1>
<p> Type a meeting name and hit +</p>
<form action=”” class=”formgroup addmeeting cf”
name=”myform”
ng-submit=”addMeeting()”
novalidate><!–ng-submit use angular js to submit a form, use a method called addMeeting, novalidate stops the browser validating instead of angular–>
<div class=”inputwrapper”>
<input type =”text” name=”meetingname”
placeholder=”Meeting name”
ng-model=”meetingname” ng-required=”true”>
</div>
<button type=”submit” class=”btn”
ng-disabled=”myform.$invalid”><!–$invalid checks a meeting name has been typed from ng-required=”true” above–>+</button>
</form>
</div>
</div><!– meetings cf –>
<div class=”card meetings cf”>
<h2>Your Meetings</h2>
<div class=”meeting” ng-repeat=”(key, meeting) in meetings”><!–ng-repeat is an angular directive that in this example pulls a key (key is the hash created in the Firebase Database) and the meeting infomation for every instance of the object (in this case the array) called ‘meetings’, created in meetings.js –>
<button class=”btn btn-delete tooltip” name=”button”
ng-click=”deleteMeeting(key)”><span>Delete this meeting</span></button> <!–ng-click executes a methood called deleteMeeting, and passes it the key (the hash) so it knows which one to delete.–>
<span class=”text”>{{meeting.name}}</span>
</div>
</div> |
So I can add more text fields to the “addMeeting()” input form, then add those input fields to the addMeeting function (these names will change). Then to display the information I just have to add more to the <span> contatining meeting.name at the bottom.
The issue with this is that the $remove command deletes the information from the database permenantly. I will be working out a way to structure the back end where a soft delete is done; having a deleted variable that changes on pressing delete, then adding a condition to the ng-repeat to not display any with the deleted variable as true.